Using Tailwind CSS with Next.js: A Comprehensive Guide
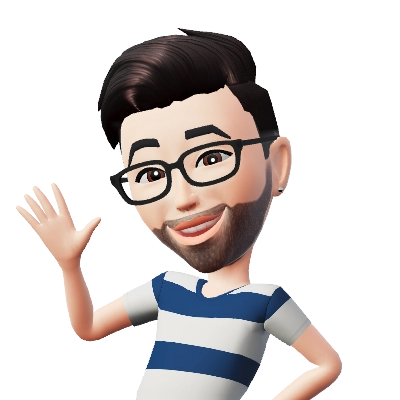
Anirban Das
4 mins read
Table of Contents
IntroductionWhy Use Tailwind CSS with Next.js?Getting StartedStep 1: Setting Up Your Next.js ProjectStep 2: Installing Tailwind CSSIntroduction
Tailwind CSS is a utility-first CSS framework that has gained immense popularity for its flexibility and ease of use. Combined with Next.js, a powerful React framework for building server-rendered applications, you can create modern, responsive web applications with minimal effort. In this blog post, we'll guide you through the steps to integrate Tailwind CSS with Next.js, ensuring a smooth development process.
Why Use Tailwind CSS with Next.js?
Before we dive into the implementation, let's briefly discuss why you might want to use Tailwind CSS with Next.js:
Utility-First Approach: Tailwind CSS allows you to build custom designs without leaving your HTML, using a set of utility classes that you can combine to create any design directly in your markup.
Responsive Design: Tailwind provides responsive design utilities, making it easy to build responsive layouts.
Customizability: With Tailwind, you can easily customize your design system by configuring the
tailwind.config.js
file.Next.js Advantages: Next.js offers features like server-side rendering, static site generation, and API routes, making it a powerful choice for modern web applications.
Getting Started
Step 1: Setting Up Your Next.js Project
First, create a new Next.js project if you don't already have one. You can do this using the following command:
npx create-next-app my-next-tailwind-app
cd my-next-tailwind-app
This will create a new directory called my-next-tailwind-app
with the default Next.js project structure.
Step 2: Installing Tailwind CSS
Next, you'll need to install Tailwind CSS and its dependencies. Run the following command:
npm install tailwindcss postcss autoprefixer
After installing Tailwind CSS, initialize it by running:
npx tailwindcss init -p
This command will create two new files in your project: tailwind.config.js
and postcss.config.js
.
Step 3: Configuring Tailwind CSS
Open the tailwind.config.js
file and configure the purge
option to remove unused styles in production. This helps keep your final CSS bundle small. Update the file to look like this:
/** @type {import('tailwindcss').Config} */
module.exports = {
content: [
'./pages/**/*.{js,ts,jsx,tsx}',
'./components/**/*.{js,ts,jsx,tsx}',
],
theme: {
extend: {},
},
plugins: [],
};
This configuration ensures that Tailwind CSS purges unused styles from all files in the pages
and components
directories.
Step 4: Adding Tailwind to Your CSS
Next, create a CSS file (e.g., styles/globals.css
) and include the following Tailwind directives:
@tailwind base;
@tailwind components;
@tailwind utilities;
Then, open the _app.js
file in the pages
directory and import the CSS file:
import '../styles/globals.css'
function MyApp({ Component, pageProps }) {
return <Component {...pageProps} />
}
export default MyApp
Building with Tailwind CSS
With Tailwind CSS set up, you can now start using its utility classes to style your components. Here’s an example of how to use Tailwind in a Next.js component.
Example Component
Create a new file called index.js
in the pages
directory and add the following code:
export default function Home() {
return (
<div className="flex items-center justify-center min-h-screen bg-gray-100">
<div className="p-8 bg-white rounded shadow-md">
<h1 className="text-2xl font-bold text-center text-gray-800">Welcome to Next.js with Tailwind CSS</h1>
<p className="mt-4 text-center text-gray-600">Get started by editing <code className="font-mono text-gray-800">pages/index.js</code></p>
</div>
</div>
)
}
In this example, we use Tailwind CSS classes to style the div
and h1
elements. The classes flex
, items-center
, justify-center
, and min-h-screen
are used to create a centered layout, while bg-gray-100
, p-8
, bg-white
, rounded
, and shadow-md
are used for styling the inner container.
Customizing Tailwind CSS
You can customize Tailwind CSS to fit your project's design requirements by editing the tailwind.config.js
file. For instance, to add custom colors or extend the default theme, you can modify the theme
section:
module.exports = {
content: [
'./pages/**/*.{js,ts,jsx,tsx}',
'./components/**/*.{js,ts,jsx,tsx}',
],
theme: {
extend: {
colors: {
customBlue: '#1DA1F2',
},
},
},
plugins: [],
};
With this configuration, you can use the new customBlue
color in your components:
<div className="text-customBlue">
This text is using a custom blue color!
</div>
Conclusion
Integrating Tailwind CSS with Next.js provides a powerful and efficient way to build modern web applications. By following the steps outlined in this guide, you can quickly set up Tailwind CSS in your Next.js project and start leveraging its utility-first approach to create responsive, customizable designs. As you continue to work with Tailwind CSS, you'll find that it significantly enhances your development workflow and allows you to create beautiful, functional UIs with ease. Happy coding!